What is Python String
Python string is the collection of the characters surrounded by single quotes, double quotes, or triple quotes. The computer does not understand the characters; internally, it stores manipulated character as the combination of the 0's and 1's.
Syntax:-
str = "Hi Python !"
Strings indexing and splitting
Like other languages, the indexing of the Python strings starts from 0. For example, The string "HELLO" is indexed as given in the below figure.
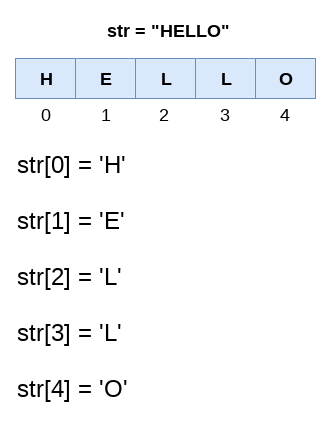
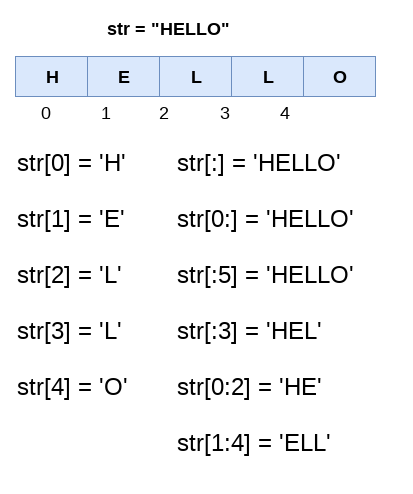
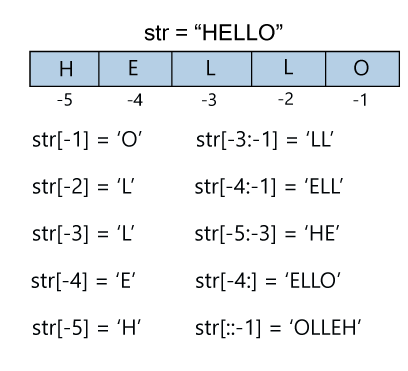
Reassigning Strings
Updating the content of the strings is as easy as assigning it to a new string. The string object doesn't support item assignment i.e., A string can only be replaced with new string since its content cannot be partially replaced. Strings are immutable in Python.
str = "HELLO"
str[0] = "h"
print(str)
it returns error
str = "HELLO"
print(str)
str = "hello"
print(str)
str[0] = "h"
print(str)
it returns error
str = "HELLO"
print(str)
str = "hello"
print(str)
Deleting the String
As we know that strings are immutable. We cannot delete or remove the characters from the string. But we can delete the entire string using the del keyword.
str = "MK Tutorial"
del str[1]
it returns error
str1 = "MK Tutorial"
del str1
print(str1)
del str[1]
it returns error
str1 = "MK Tutorial"
del str1
print(str1)
String Operators
Operator | Description |
---|---|
+ | It is known as concatenation operator used to join the strings given either side of the operator. |
* | It is known as repetition operator. It concatenates the multiple copies of the same string. |
[] | It is known as slice operator. It is used to access the sub-strings of a particular string. |
[:] | It is known as range slice operator. It is used to access the characters from the specified range. |
in | It is known as membership operator. It returns if a particular sub-string is present in the specified string |
not in | It is also a membership operator and does the exact reverse of in. It returns true if a particular substring is not present in the specified string. |
r/R | It is used to specify the raw string. Raw strings are used in the cases where we need to print the actual meaning of escape characters such as "C://python". To define any string as a raw string, the character r or R is followed by the string. |
% | It is used to perform string formatting. It makes use of the format specifiers used in C programming like %d or %f to map their values in python. We will discuss how formatting is done in python. |
Example:-
str = "Hello"
str1 = " world"
print(str*3) # prints HelloHelloHello
print(str+str1)# prints Hello world
print(str[4]) # prints o
print(str[2:4]); # prints ll
print('w' in str) # prints false as w is not present in str
print('wo' not in str1) # prints false as wo is present in str1.
print(r'C://python37') # prints C://python37 as it is written
print("The string str : %s"%(str)) # prints The string str : Hello
str1 = " world"
print(str*3) # prints HelloHelloHello
print(str+str1)# prints Hello world
print(str[4]) # prints o
print(str[2:4]); # prints ll
print('w' in str) # prints false as w is not present in str
print('wo' not in str1) # prints false as wo is present in str1.
print(r'C://python37') # prints C://python37 as it is written
print("The string str : %s"%(str)) # prints The string str : Hello
Python String functions
Method | Description |
---|---|
capitalize() | It capitalizes the first character of the String. This function is deprecated in python3
Example:- str = "mktutorial" str2 = str.capitalize() print(str2) It returns Mktutorial |
center(width) | It returns a space padded string with the original string centred with equal number of left and right spaces. Example:- str = "MKTutorial" str2 = str.center(20) print(str2) It returns MKTUTORIAL |
count(string) | It counts the number of occurrences of a substring in a String between begin and end index. Example:- str = "MKTutorial" str2 = str.count('t') print(str2) It returns 1 |
endswith(suffix) | It returns a Boolean value if the string terminates with given suffix between begin and end. Example:- str = "MKTutorial." isends = str.endswith(".") print(isends) It returns True |
find(substring) | It returns the index value of the string where substring is found between begin index and end index. Example:- str = "MK Tutorial" substring = "Tutorial" index = str.find(substring) print(index) It returns 3 |
index(subsring) | It throws an exception if string is not found. It works same as find() method. Example:- str = "Welcome to the MK Tutorial" str2 = str.index("to") print(str2) It returns 20 |
isalnum() | It returns true if the characters in the string are alphanumeric i.e., alphabets or numbers and there is at least 1 character. Otherwise, it returns false. Example:- str = "Welcome to the MK Tutorial" str2 = str.isalnum() print(str2) It returns True |
isalpha() | It returns true if all the characters are alphabets and there is at least one character, otherwise False. Example:- str = "Welcome to the MK Tutorial" str2 = str.isalpha() print(str2) It returns True |
isdecimal() | It returns true if all the characters of the string are decimals. Example:- str = "Welcome to the MK Tutorial" str2 = str.isdecimal() print(str2) It returns False |
isdigit() | It returns true if all the characters are digits and there is at least one character, otherwise False. Example:- str = "Welcome to the MK Tutorial" str2 = str.isdigit() print(str2) It returns False |
islower() | It returns true if the characters of a string are in lower case, otherwise false. Example:- str = "Welcome to the MK Tutorial" str2 = str.islower() print(str2) It returns False |
isnumeric() | It returns true if the string contains only numeric characters. Example:- str = "Welcome to the MK Tutorial" str2 = str.isnumeric() print(str2) It returns False |
isupper() | It returns false if characters of a string are in Upper case, otherwise False. Example:- str = "Welcome to the MK Tutorial" str2 = str.isupper() print(str2) It returns False |
isspace() | It returns true if the characters of a string are white-space, otherwise false. Example:- str = "Welcome to the MK Tutorial" str2 = str.isspace() print(str2) It returns False |
len(string) | It returns the length of a string. Example:- str = "MK Tutorial" n=len(str) print(n) It returns 11 |
lower() | It converts all the characters of a string to Lower case. Example:- str = "MK Tutorial" str = str.lower() print(str) It returns mk tutorial |
lstrip() | It removes all leading whitespaces of a string and can also be used to remove particular character from leading. Example:- str = "MK Tutorial" str2 = str.lstrip() print(str2) It returns MK Tutorial |
replace(old,new[,count]) | It replaces the old sequence of characters with the new sequence. The max characters are replaced if max is given. Example:- str = "MK Tutorial" str2 = str.replace("MK","IT") print(str2) It returns IT Tutorial |
rfind(str,beg=0,end=len(str)) | It is similar to find but it traverses the string in backward direction. Example:- str = "MK Tutorial" str2 = str.rfind("t") print(str2) It returns 5 |
rindex(str,beg=0,end=len(str)) | It is same as index but it traverses the string in backward direction. Example:- str = "MK Tutorial" str2 = str.rindex("t") print(str2) It returns 5 |
split(str,num=string.count(str)) | Splits the string according to the delimiter str. The string splits according to the space if the delimiter is not provided. It returns the list of substring concatenated with the delimiter. Example:- str = "MK Tutorial" str2 = str.split(" ") print(str2) It returns ["MK","Tutorial"] |
startswith(str,beg=0,end=len(str)) | It returns a Boolean value if the string starts with given str between begin and end. Example:- str = "MK Tutorial" str2 = str.startswith("M") print(str2) It returns True |
upper() | It converts all the characters of a string to Upper Case. Example:- str = "MK Tutorial" str2 = str.upper() print(str2) It returns MK TUTORIAL |