Datatypes in Python
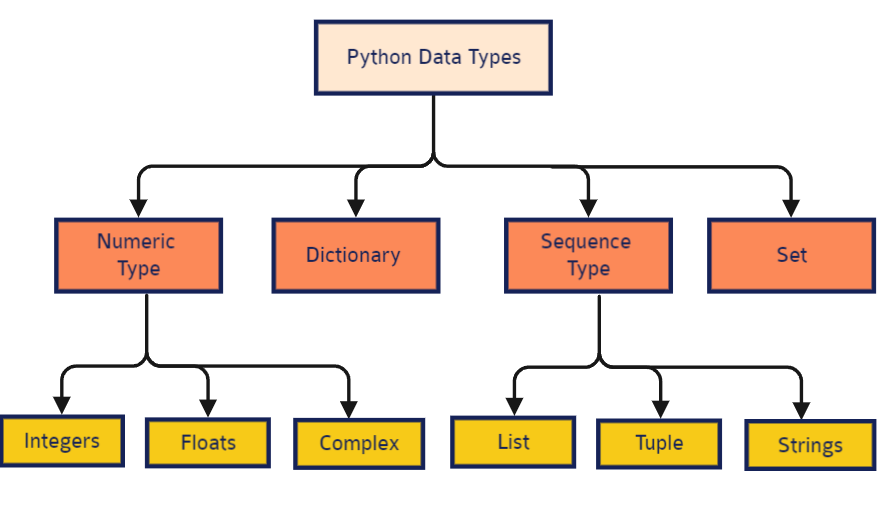
What is List?
Lists are used to store multiple items in a single variable.Lists are created using square brackets and if we want to take input from user in list format then we will use list keyword
thislist = ["apple", "banana", "cherry"]
print(thislist)
print(thislist)
Characterstics of List
- List items are ordered, changeable, and allow duplicate values.List items are indexed, the first item has index [0], the second item has index [1] etc.
- When we say that lists are ordered, it means that the items have a defined order, and that order will not change.If you add new items to a list, the new items will be placed at the end of the list.
- Since lists are indexed, lists can have items with the same value:
- The list is changeable, meaning that we can change, add, and remove items in a list after it has been created.
List Items - Data Types
List items can be of any data type:
list1 = ["apple", "banana", "cherry"]
list2 = [1, 5, 7, 9, 3]
list3 = [True, False, False]
list4 = ["abc", 34, True, 40, "male"]
list2 = [1, 5, 7, 9, 3]
list3 = [True, False, False]
Ordered List Checking
a = [ 1, 2, "Ram", 3.50, "Rahul", 5, 6 ]
b = [ 1, 2, 5, "Ram", 3.50, "Rahul", 6 ]
a == b
It returns false because a and b order is not same
a = [ 1, 2, "Ram", 3.50, "Rahul", 5, 6]
b = [ 1, 2, "Ram", 3.50, "Rahul", 5, 6]
a == b
It returns true because a and b order is same
b = [ 1, 2, 5, "Ram", 3.50, "Rahul", 6 ]
a == b
It returns false because a and b order is not same
a = [ 1, 2, "Ram", 3.50, "Rahul", 5, 6]
b = [ 1, 2, "Ram", 3.50, "Rahul", 5, 6]
a == b
It returns true because a and b order is same
List Indexing and Splitting
list_varible(start:stop:step)
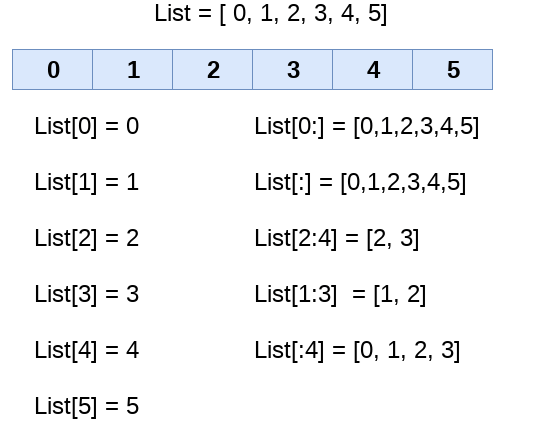
If we write print(list[1:6:2]) then it returns [1,3,5]
List Forward and Backward Direction
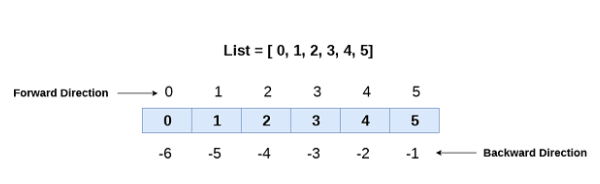
list = [1,2,3,4,5]
print(list[-1]) It returns [5]
print(list[-3:]) It returns [3,4,5]
print(list[:-1]) It returns [1,2,3,4]
print(list[-3:-1]) It returns [3,4]
print(list[-1]) It returns [5]
print(list[-3:]) It returns [3,4,5]
print(list[:-1]) It returns [1,2,3,4]
print(list[-3:-1]) It returns [3,4]
Updating List Values
We can also update the value in list directly
list = [1, 2, 3, 4, 5, 6]
print(list) It returns [1, 2, 3, 4, 5, 6]
list[2] = 10
print(list) It returns [1, 2, 10, 4, 5, 6]
list[1:3] = [89, 78]
print(list) It returns [1, 89, 78, 4, 5, 6]
list[-1] = 25
print(list) It returns [1, 89, 78, 4, 5, 25]
print(list) It returns [1, 2, 3, 4, 5, 6]
list[2] = 10
print(list) It returns [1, 2, 10, 4, 5, 6]
list[1:3] = [89, 78]
print(list) It returns [1, 89, 78, 4, 5, 6]
list[-1] = 25
print(list) It returns [1, 89, 78, 4, 5, 25]
Python List Operations
The concatenation (+) and repetition (*) operators work in the same way as they were working with the strings. The different operations of list are
- Repetition
- Concatenation
- Length
- Iteration
- Membership
list1 = [12, 14, 16, 18, 20]
l = list1 * 2
print(l)
It returns [12, 14, 16, 18, 20, 12, 14, 16, 18, 20]
list1 = [12, 14, 16, 18, 20]
list2 = [9, 10, 32, 54, 86]
l = list1 + list2
print(l)
It returns [12, 14, 16, 18, 20, 9, 10, 32, 54, 86]
list1 = [12, 14, 16, 18, 20, 23, 27, 39, 40]
len(list1)
It returns 9
list1 = [12, 14, 16, 39, 40]
for i in list1:
print(i)
It returns 12
14
16
39
40
list1 = [100, 200, 300, 400, 500] print(300 in list1) It returns True
print(600 in list1) It returns False
List Functions
Method | Description |
---|---|
append() | Adds an element at the end of the list Example:- fruits = ['apple', 'banana', 'cherry'] fruits.append("orange") print(fruits) It returns ['apple', 'banana', 'cherry', 'orange'] |
clear() | Removes all the elements from the list Example:- fruits = ['apple', 'banana', 'cherry', 'orange'] fruits.clear() print(fruits) It returns [] |
copy() | Returns a copy of the list Example:- fruits = ['apple', 'banana', 'cherry', 'orange'] x = fruits.copy() print(x) It returns ['apple', 'banana', 'cherry','orange'] |
count() | Returns the number of elements with the specified value Example:- fruits = ['apple', 'banana', 'cherry'] x = fruits.count("cherry") print(x) It returns 1 |
extend() | Add the elements of a list (or any iterable), to the end of the current list Example:- fruits = ['apple', 'banana', 'cherry'] cars = ['Ford', 'BMW', 'Volvo'] fruits.extend(cars) print(fruits) It returns ['apple', 'banana', 'cherry', 'Ford', 'BMW', 'Volvo'] |
index() | Returns the index of the first element with the specified value Example:- fruits = ['apple', 'banana', 'cherry'] x = fruits.index("cherry") print(x) It returns 2 |
insert() | Adds an element at the specified position Example:- fruits = ['apple', 'banana', 'cherry'] fruits.insert(1, "orange") print(fruits) It returns ['apple', 'orange', 'banana', 'cherry'] |
pop() | Removes the element at the specified position Example:- fruits = ['apple', 'banana', 'cherry'] fruits.pop(1) print(fruits) It returns ['apple','cherry'] |
remove() | Removes the first item with the specified value Example:- fruits = ['apple', 'banana', 'cherry'] fruits.remove("banana") print(fruits) It returns ['apple','cherry'] |
reverse() | Reverses the order of the list Example:- fruits = ['apple', 'banana', 'cherry'] fruits.reverse() print(fruits) It returns ['cherry', 'banana', 'apple'] |
Sort() | Sorts the list Example:- cars = ['Ford', 'BMW', 'Volvo'] cars.sort() print(cars) It returns ['BMW', 'Ford', 'Volvo'] |
len() | Length of the list Example:- cars = ['Ford', 'BMW', 'Volvo'] x=len(cars) print(x) It returns 3 |
max() | Largest number in the list Example:- list1 = [1,2,3,4,5,6] x=max(list1) print(x) It returns 6 |
min() | Minimum number in the list Example:- list1 = [1,2,3,4,5,6] x=min(list1) print(x) It returns 1 |