Python Classes and Objects
Python is an object oriented programming language.Almost everything in Python is an object, with its properties and methods
A Class is like an object constructor, or a "blueprint" for creating objects.
Create a Class
To create a class, use the keyword class:
Example:-
class MyClass:
x = 5
x = 5
Create Object
Now we can use the class named MyClass to create objects:
Example:-
p1 = MyClass()
print(p1.x)
print(p1.x)
The __init__() Function
The examples above are classes and objects in their simplest form, and are not really useful in real life applications.
To understand the meaning of classes we have to understand the built-in __init__() function.All classes have a function called __init__(), which is always executed when the class is being initiated.Use the __init__() function to assign values to object properties, or other operations that are necessary to do when the object is being created:
Instance method in Python
Instance method performs a set of actions on the data/value provided by the instance variables. If we use instance variables inside a method, such methods are called instance methods.
Class method in Python
Class method is method that is called on the class itself, not on a specific object instance. Therefore, it belongs to a class level, and all class instances share a class method.
Static method in Python
Static method is a general utility method that performs a task in isolation. This method doesn’t have access to the instance and class variable.
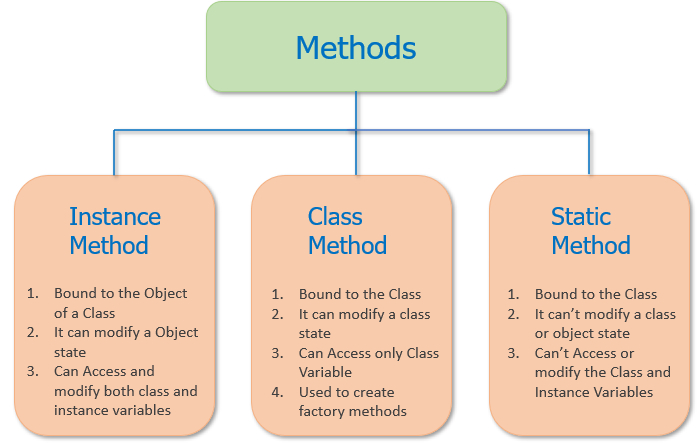
Example:-
class aptech:
def __init__(self):
print("Hello aptech")
def display1(self):
print("instance method") #instance method
def display2():
print("class method") #class method
def display3(cls):
print("static method") #static method
ap=aptech()
ap.display1() #instance method called by object
aptech.display2() #class method called by class
ap.display3() #static method also calle by object
def __init__(self):
print("Hello aptech")
def display1(self):
print("instance method") #instance method
def display2():
print("class method") #class method
def display3(cls):
print("static method") #static method
ap=aptech()
ap.display1() #instance method called by object
aptech.display2() #class method called by class
ap.display3() #static method also calle by object