Effects in jQuery
jQuery enables us to add effects on a web page. jQuery effects can be categorized into fading, sliding, hiding/showing and animation effects.
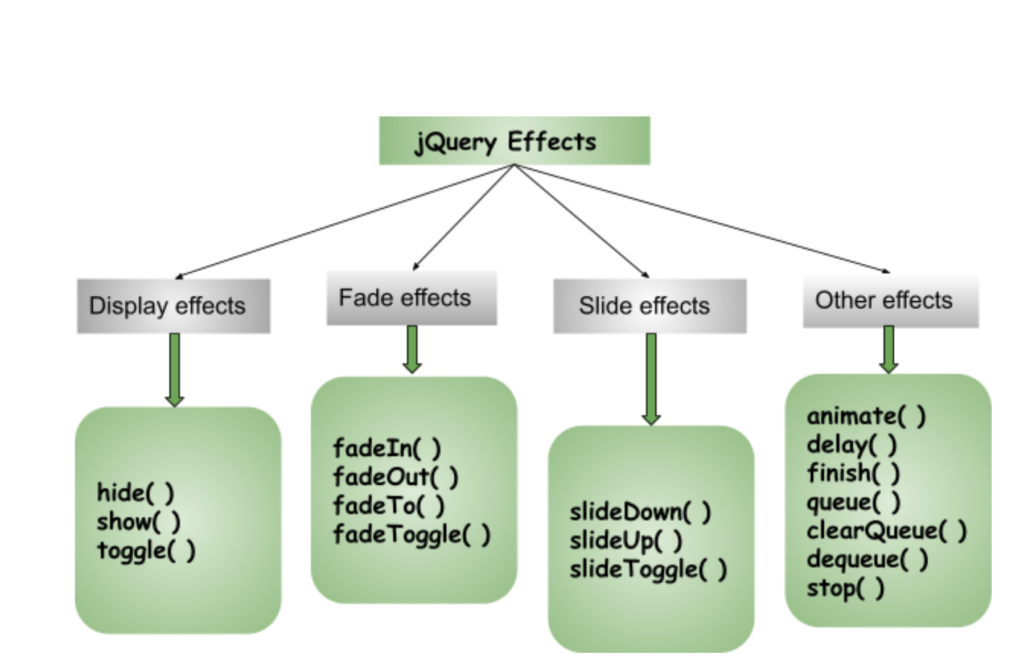
jQuery hide() and show() effect
<html lang = "en"><head>
<title> jQuery </title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"> </script>
<script>
$(document).ready(function(){
$("#hide").click(function(){
$("p").hide();
});
$("#show").click(function(){
$("p").show();
});
});
</script>
</head>
<body>
<p>If you click on the "Hide" button, I will disappear.</p>
<button id="hide">Read More</button> <button id="show">less</button> </body>
</html>
Output
If you click on the "Hide" button, I will disappear.
The optional speed parameter specifies the speed of the hiding/showing, and can take the following values: "slow", "fast", or milliseconds.
$(selector).hide(speed,callback);
$(selector).show(speed,callback);
The optional callback parameter is a function to be executed after the hide() or show() method completes (you will learn more about callback functions in a later chapter).
<head>
<title> jQuery </title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"> </script>
<script>
$(document).ready(function(){ $("button").click(function(){ $("p").hide(1000); }); }); </script>
</head>
<body>
<button id="hide">hide</button> <p>This is a paragraph with little content.</p>
<p>This is another small paragraph.</p>
</body>
</html>
OUTPUT
This is a paragraph with little content.
This is another small paragraph.
jQuery toggle()
You can also toggle between hiding and showing an element with the toggle() method.Shown elements are hidden and hidden elements are shown:
<html lang = "en">
<head>
<title> jQuery </title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"> </script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("p").toggle();
}); }); </script>
</head>
<body>
<button >Toggle between hiding and showing the paragraph</button>
<p>This is a paragraph with little content.</p>
<p>This is another small paragraph..</p>
</body>
</html>
This is a paragraph with little content.
This is another small paragraph..
jQuery FADE EFFECT
With jQuery you can fade an element in and out of visibility.
jQuery has the following fade methods:
- fadeIn()
- fadeOut()
- fadeToggle()
- fadeTo()
jQuery fadeIn() method
The jQuery fadeIn() method is used to fade in a hidden element.<html lang = "en">
<head>
<title> jQuery </title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"> </script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#div1").fadeIn();
$("#div2").fadeIn("slow");
$("#div3").fadeIn(3000);
}); }); </script>
</head>
<body>
<p>Demonstrate fadeIn() with different parameters.</p> <button >Click to fade in boxes</button>
<div id="div1" style="width:80px;height:80px;display:none;background-color:red;"></div>
<div id="div2" style="width:80px;height:80px;display:none;background-color:green;"></div>
<div id="div3" style="width:80px;height:80px;display:none;background-color:blue;"></div> </body>
</html>
fade out() method
The jQuery fadeOut() method is used to fade out a visible element.
<html lang = "en"><head>
<title> jQuery </title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"> </script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#div1").fadeOut();
$("#div2").fadeOut("slow");
$("#div3").fadeOut(3000);
}); }); </script>
</head>
<body>
<p>Demonstrate fadeIn() with different parameters.</p> <button >Click to fade in boxes</button>
<div id="div1" style="width:80px;height:80px;background-color:red;"></div>
<div id="div2" style="width:80px;height:80px;background-color:green;"></div>
<div id="div3" style="width:80px;height:80px;background-color:blue;"></div> </body>
</html>
fade toggle() method
The jQuery fadeToggle() method toggles between the fadeIn() and fadeOut() methods.
If the elements are faded out, fadeToggle() will fade them in.
If the elements are faded in, fadeToggle() will fade them out.
<head>
<title> jQuery </title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"> </script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#div1").fadeToggle();
$("#div2").fadeToggle("slow");
$("#div3").fadeToggle(3000);
}); }); </script>
</head>
<body>
<p>Demonstrate fadeIn() with different parameters.</p> <button >Click to fade in boxes</button>
<div id="div1" style="width:80px;height:80px;background-color:red;"></div>
<div id="div2" style="width:80px;height:80px;background-color:green;"></div>
<div id="div3" style="width:80px;height:80px;background-color:blue;"></div> </body>
</html>
jQuery fadeTo() Method
<html lang = "en"><head>
<title> jQuery </title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"> </script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#div1").fadeTo("slow", 0.15);
$("#div2").fadeTo("slow", 0.4);
$("#div3").fadeTo("slow", 0.7);
}); }); </script>
</head>
<body>
<p>Demonstrate fadeIn() with different parameters.</p> <button >Click to fade in boxes</button>
<div id="div1" style="width:80px;height:80px;background-color:red;"></div>
<div id="div2" style="width:80px;height:80px;background-color:green;"></div>
<div id="div3" style="width:80px;height:80px;background-color:blue;"></div> </body>
</html>
jQuery Sliding Methods
With jQuery you can create a sliding effect on elements.
jQuery has the following slide methods:
- slideDown()
- slideUp()
- slideToggle()
<head>
<title> jQuery </title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"> </script>
<script>
$(document).ready(function(){
$("#flip").click(function(){
$("#panel").slideToggle("slow");
});
});
</script>
<style>
#panel, #flip {
padding: 5px;
text-align: center;
background-color: #e5eecc;
border: solid 1px #c3c3c3;
}
#panel {
padding: 50px;
display: none;
}
</style>
</head>
<body>
<div id="flip">Click to slide the panel down or up</div>
<div id="panel">Hello World</div>
</body>
</html>
jQuery Animations - The animate() Method
The jQuery animate() method is used to create custom animations.
<html lang = "en"><head>
<title> jQuery </title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"> </script>
<script>
$(document).ready(function(){
$("button").click(function(){
var div = $("div");
div.animate({left: '100px'}, "slow");
div.animate({fontSize: '3em'}, "slow");
});
});
</script>
</head>
<body>
<button >Start Animation</button>
<div style="background:#98bf21;height:100px;width:200px;position:absolute;">HELLO</div> </body>
</html>
jQuery stop() Method
The jQuery stop() method is used to stop an animation or effect before it is finished.
The stop() method works for all jQuery effect functions, including sliding, fading and custom animations.
<html lang = "en">
<head>
<title> jQuery </title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"> </script>
<script>
$(document).ready(function(){
$("#flip").click(function(){
$("#panel").slideDown(5000);
});
$("#stop").click(function(){
$("#panel").stop();
});
});
</script>
<style>
#panel, #flip {
padding: 5px;
font-size: 18px;
text-align: center;
background-color: #555;
color: white;
border: solid 1px #666;
border-radius: 3px;
}
#panel {
padding: 50px;
display: none;
}
</style>
</head>
<body>
<button id="stop">Stop sliding</button> <div id="flip">Click to slide down panel</div> <div id="panel">Hello world!</div> </body>
</html>
jQuery Callback Functions
JavaScript statements are executed line by line. However, with effects, the next line of code can be run even though the effect is not finished. This can create errors.
To prevent this, you can create a callback function.
A callback function is executed after the current effect is finished.
Typical syntax: $(selector).hide(speed,callback);
<html lang = "en">
<head>
<title> jQuery </title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"> </script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("p").hide("slow", function(){
alert("The paragraph is now hidden");
});
});
});
</script>
</head>
<body>
<p>This is a paragraph with little content.</p>
<button id="hide">HIDE</button> </body>
</html>