JavaScript Object
Objects are properties and methods and resemble to real life objects.properties specify the characteristics or attributes of an object,while methods identify the behavior of an object.for example car,pen,table
- JavaScript is an object-based language. Everything is an object in JavaScript.
- JavaScript is template based not class based. Here, we don't create class to get the object. But, we direct create objects.
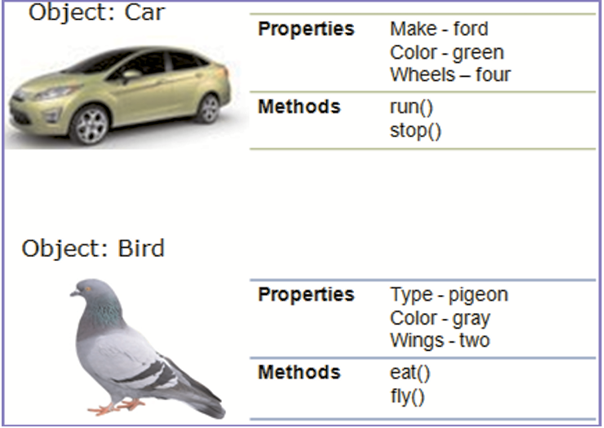
Two type of Object
1)Custom Objects2)Built-in Objects
1)Custom Object
Three Ways Creating Custom Objects in JavaScript
- By object literal
- By creating instance of Object directly (using new keyword)
- By using an object constructor (using new keyword)
Example-By object literal
<html><body>
<script>
emp={id:102,name:"Riya",salary:40000}
document.write(emp.id+" "+emp.name+" "+emp.salary);
</script>
</body>
</html>
Example-By creating instance of Object
<html><body>
<script>
var emp=new Object();
emp.id=101;
emp.name="Ravi Malik";
emp.salary=50000;
document.write(emp.id+" "+emp.name+" "+emp.salary);
</script>
</body>
</html>
Example-By using an object constructor
<html><body>
<script>
function emp(id,name,salary)
{
this.id=id;
this.name=name;
this.salary=salary;
}
e=new emp(103,"Riya",30000);
document.write(e.id+" "+e.name+" "+e.salary);
</script>
</body>
</html>
2) Built-in Object
The built-in Objects are static objects which can be used to extend the functionality in the script...........for example
- String Object
- Math Object
- Date Object
- Window Object
- History Object
- Navigator Object
- Document Object
<html>
<body>
<script>
var full_name=new String("aptech student");
document.write("no. of characrter:"+full_name.length+"<br>")
document.write("no. of characrter:"+full_name.charAt(2)+"<br>")
document.write("no. of characrter:"+full_name.split("",5)+"<br>")
document.write("no. of characrter:"+full_name.toUpperCase()+"<br>")
/*date object*/
var today=new Date()
document.write(today.getDate()+"<br>")
document.write(today.getMonth()+"<br>")
document.write(today.getFullYear()+"<br>")
</script>
</body>
</html>
document.getElementById
<html><head>
<script>
function getcube()
{
var number=document.getElementById("number").value;
//alert(number*number*number);
document.write(number*number*number);
}
</script>
</head>
<body>
<form>
Enter No:<input type="text" id="number" name="number"/><br>
<input type="button" value="cube" onclick="getcube()"/>
</form>
</body>
</html>
document.getElementByClass
<html><body>
<div class="example">
A div element with class="example"
</div>
<div class="example">
A div element with class="example"
</div>
<p class="example">
A p element with class="example"
</p>
<p>The number of elements with class="example" is:</p>
<p id="demo"></p>
<script>
var numb = document.getElementsByClassName("example").length;
document.getElementById("demo").innerHTML = numb;
</script>
</body>
</html>