What is MVC
AngularJS support MVC architecture in software devlopment.MVC stands for Model View Controller. It is a software design pattern for developing web applications.
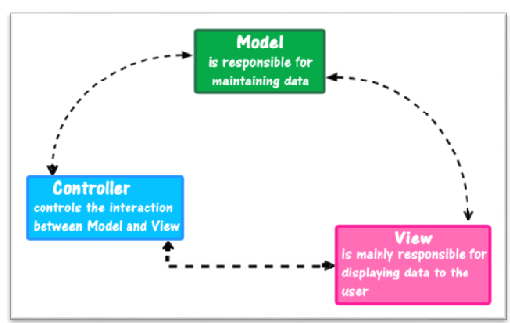
MODEL:The Model holds the data and logic
VIEW:The view holds the visual layout and presentation
CONTROLLER:Controller coordinates the model and view.
For easy understanding,you can think of model and view as two persons standing on two sides of a bridge and the controller as the bridge which connects these two persons.
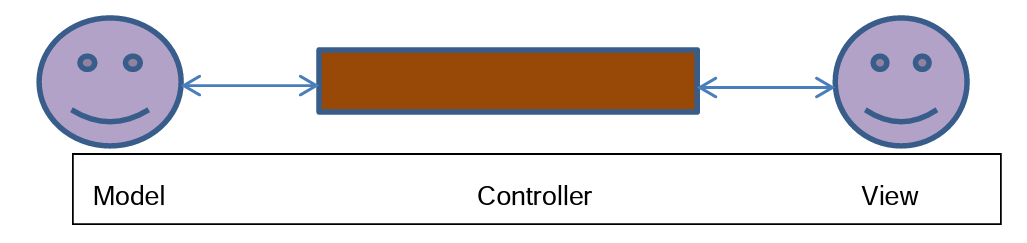
before creating actual Hello World ! application using AngularJS, let us see the parts of a AngularJS application. An AngularJS application consists of following three important parts −
- ng-app − This directive defines and links an AngularJS application to HTML.
- ng-model − This directive binds the values of AngularJS application data to HTML input controls.
- ng-bind − This directive binds the AngularJS Application data to HTML tags.
Introduction to a simple AngularJS App
Example-1
<html lang="en"><head>
<title>AngularJS First Application</title>
</head>
<body>
<h2>Sample Application</h2>
<div ng-app = "">
<p>Enter your Name: <input type = "text" ng-model = "name"></p>
<p>Hello <span ng-bind = "name"></span></p>
</div>
<script src = "https://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js">
</script>
</body>
</html>
Example-2
<html lang="en"><head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.5/angular.min.js"></script>
</head>
<body ng-app="myapp">
<div ng-controller="HelloController" >
<h2>Hello {{helloTo.title}} !</h2>
</div>
<script>
angular.module("myapp", [])
.controller("HelloController", function($scope) {
$scope.helloTo = {};
$scope.helloTo.title = "World, AngularJS";
} );
</script>
</body>
</html>
ng-app directives
ng-app: This directive starts an AngularJS Application.
It is required to tell which part of HTML contains the AngularJS app. You can do this by adding the ng-app attribute to the root HTML element of the AngularJS app. You can either add it to the html element or the body element
View Part
<div ng-controller="HelloController" ><h2>Hello {{helloTo.title}} !</h2>
</div>
Controller Part
<script>angular.module("myapp", [])
.controller("HelloController", function($scope) {
$scope.helloTo = {};
$scope.helloTo.title = "World, AngularJS";
} );
</script>
How AngularJS Integrates with HTML
- The ng-app:- directive indicates the start of AngularJS application.
- The ng-model:- directive creates a model variable named name, which can be used with the HTML page and within the div having ng-app directive.
- The ng-bind:- then uses the name model to be displayed in the HTML tag whenever user enters input in the text box.
- Closing</div>tag indicates the end of AngularJS application.