What is Operators and Operands
x+y=z in this expression x,y and z is operands and + and = is an operator
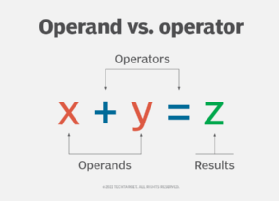
Types of Operands
- Unary (a):-Unary operators are the operators that perform operations on a single operand to produce a new value
- Binary (a+b):-A binary operator is an operator that operates on two operands and manipulates them to return a result
- Ternary (a+b+c+d):-A Ternary operator is an operator that operates on three or more operands and manipulates them to return a result
Types of Operators
Arthmetic Operator
- + (Plus)
- - (Minus)
- * (Multiply)
- / (Divide)
- % (Mod)
Relational Operator
- > (Greater then)
- < (Less then)
- >= (Greater then equal to)
- <= (Less then equal to)
- = (Equal to)
- == (Equal equals)
Logical Operator
- && (And):-The logical AND operator is an operator that performs a logical conjunction on two statements. It only yields a value of “true” when both statements are true. If one of the two statements is false, then the logical AND operator yields a “false” value.
- || (Or):-The OR operator is a Boolean operator which would return the value TRUE if one or more conditions is true
- ! (Not):-An operation on logical values that changes true to false, and false to true
Assignment (Shorthand) Operator
- += (Plus equal)
- -= (Minus equal)
- *= (Multiply equal)
- /= (Divide equal)
- %= (Mod equal)
Bitwise Operator
- >> (Right Shift)
- << (Left Shift)
- ^ (Power)
- ~ (Destructor)
Sizeof Operator
- sizeof:-Sizeof is a much-used operator in the C. It is a compile-time unary operator which can be used to compute the size of its operand
#include<stdio.h>
#include<conio.h>
void main()
{
int a;
clrscr();
printf("%d",sizeof(a));
getch();
}
#include<conio.h>
void main()
{
int a;
clrscr();
printf("%d",sizeof(a));
getch();
}
Conditional Operator
- ? (If)and(else):-The conditional operator is also known as a ternary operator. The conditional statements are the decision-making statements which depends upon the output of the expression. It is represented by two symbols, i.e., '?' and ':'.
#include<stdio.h>
#include<conio.h>
void main()
{
int age;
clrscr();
printf("Enter age=");
scanf("%d",&age);
//printf("%d",age>=18?1:0);
printf("%s",age>=18?"Eligible":"Not Eligible");
getch();
}
#include<conio.h>
void main()
{
int age;
clrscr();
printf("Enter age=");
scanf("%d",&age);
//printf("%d",age>=18?1:0);
printf("%s",age>=18?"Eligible":"Not Eligible");
getch();
}
Increment/Decrement Operator
- ++ (Increment)and -- (Decrement):-Increment Operator is used to increase the value of the operand by 1 whereas the Decrement Operator is used to decrease the value of the operand by 1.
Increment and Decrement operators is basically two of types
- Prefix (++a):-The prefix increment operator (++) adds one to its operand before print
- Postfix (a++):-The prefix increment operator (++) adds one to its operand after print